1. API References
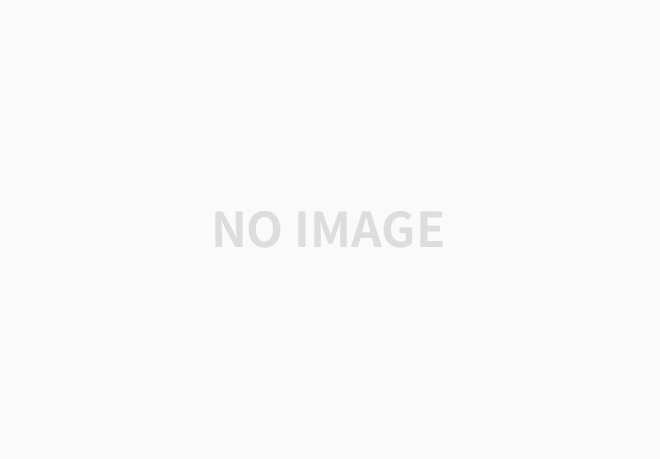
2. Source code 적용
2-1. Dependency
- build.gradle
buildscript {
ext {
springBootVersion = '1.5.8.RELEASE'
}
repositories {
mavenCentral()
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}")
}
}
apply plugin: 'java'
apply plugin: 'eclipse'
apply plugin: 'org.springframework.boot'
group = 'com.linebot.springboot'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
jar {
manifest {
attributes 'Title': 'Line bot test', 'Main-Class': 'com.linebot.springboot.LineBotSpringBootApplication'
}
dependsOn configurations.runtime
from {
configurations.compile.collect {it.isDirectory()? it: zipTree(it)}
}
}
dependencies {
compile('org.springframework.boot:spring-boot-starter-data-jpa')
compile('org.springframework.boot:spring-boot-starter-web')
compile('org.springframework.boot:spring-boot-starter-tomcat')
// line bot spring boot
compile('com.linecorp.bot:line-bot-spring-boot:1.10.0')
// lombok
compileOnly ('org.projectlombok:lombok:1.16.18')
compile group: 'org.apache.commons', name: 'commons-lang3', version: '3.6'
// crawling
compile('org.jsoup:jsoup:1.8.1')
runtime('org.hsqldb:hsqldb')
// MySQL DB
runtime('mysql:mysql-connector-java')
// Mongo DB
compile('org.springframework.boot:spring-boot-starter-data-mongodb')
testCompile('org.springframework.boot:spring-boot-starter-test')
// Google Cloud Vision API
compile 'com.google.cloud:google-cloud-vision:0.25.0-beta'
}
2-2. Controlller
- GoogleVisionController
package com.linebot.springboot.controller;
import com.google.cloud.vision.v1.*;
import com.google.protobuf.ByteString;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
@Controller
@RequestMapping(value = "/vision")
public class GoogleVisionController {
private static final String BASE_PATH = "/Applications/ssingssing2/ocr/";
@RequestMapping(value = "/analysis")
@ResponseBody
public boolean analysisImageToString (
@RequestParam(value = "fileName") String fileName) throws Exception {
// Instantiates a client
try (ImageAnnotatorClient vision = ImageAnnotatorClient.create()) {
// The path to the image file to annotate
//String fileName = "./resources/wakeupcat.jpg";
// Reads the image file into memory
Path path = Paths.get(BASE_PATH + fileName);
byte[] data = Files.readAllBytes(path);
ByteString imgBytes = ByteString.copyFrom(data);
// Builds the image annotation request
List<AnnotateImageRequest> requests = new ArrayList<>();
Image img = Image.newBuilder().setContent(imgBytes).build();
Feature feat = Feature.newBuilder().setType(Feature.Type.TEXT_DETECTION).build();
AnnotateImageRequest request = AnnotateImageRequest.newBuilder()
.addFeatures(feat)
.setImage(img)
.build();
requests.add(request);
// Performs label detection on the image file
BatchAnnotateImagesResponse response = vision.batchAnnotateImages(requests);
List<AnnotateImageResponse> responses = response.getResponsesList();
for (AnnotateImageResponse res : responses) {
if (res.hasError()) {
System.out.printf("Error: %s\n", res.getError().getMessage());
return false;
}
for (EntityAnnotation annotation : res.getTextAnnotationsList()) {
annotation.getAllFields().forEach((k, v)->
System.out.printf("%s : %s\n", k, v.toString()));
}
}
}
return true;
}
}
2-3. Test
$ ls -ll /Applications/ssingssing2/ocr/
total 40
-rw-r--r--@ 1 ssingssing2 staff 5969 10 27 15:04 googlelogo_color_272x92dp.png
-rw-r--r--@ 1 ssingssing2 staff 7108 10 27 15:03 googlelogo_light_color_272x92dp.png
-rw-r--r--@ 1 ssingssing2 staff 2317 10 26 18:40 ocr-java-test-97a423949d58.json
1) http://localhost:8080/vision/analysis?fileName=googlelogo_light_color_272x92dp.png
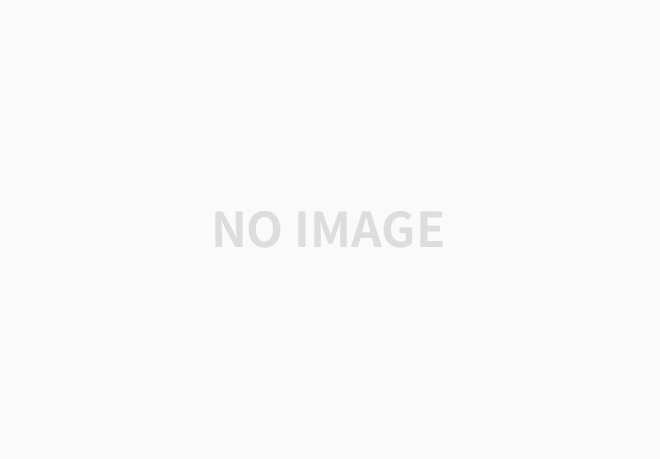
2017-10-27 15:15:56.753 DEBUG 28013 --- [nio-8080-exec-5] o.s.b.w.f.OrderedRequestContextFilter : Bound request context to thread: org.apache.catalina.connector.RequestFacade@28d1a4b7
google.cloud.vision.v1.EntityAnnotation.locale : en
google.cloud.vision.v1.EntityAnnotation.description : Google
google.cloud.vision.v1.EntityAnnotation.bounding_poly : vertices {
x: 3
y: 3
}
vertices {
x: 534
y: 3
}
vertices {
x: 534
y: 178
}
vertices {
x: 3
y: 178
}
google.cloud.vision.v1.EntityAnnotation.description : Google
google.cloud.vision.v1.EntityAnnotation.bounding_poly : vertices {
x: 3
y: 3
}
vertices {
x: 534
y: 3
}
vertices {
x: 534
y: 178
}
vertices {
x: 3
y: 178
}
2017-10-27 15:15:57.685 DEBUG 28013 --- [nio-8080-exec-5] o.s.b.w.f.OrderedRequestContextFilter : Cleared thread-bound request context: org.apache.catalina.connector.RequestFacade@28d1a4b7
2) http://localhost:8080/vision/analysis?fileName=googlelogo_color_272x92dp.png
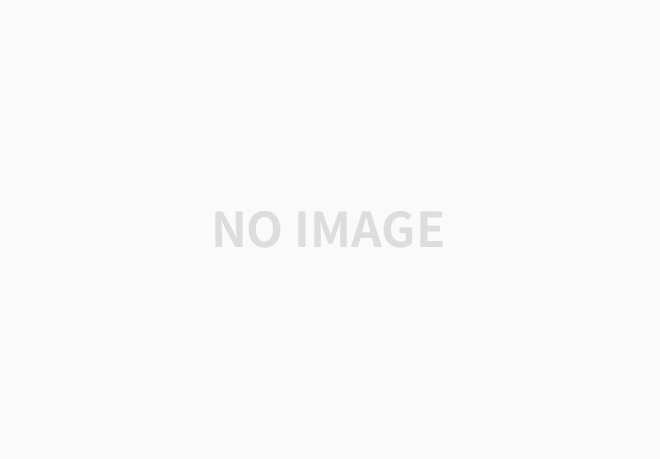
2017-10-27 15:15:30.730 DEBUG 28013 --- [nio-8080-exec-3] o.s.b.w.f.OrderedRequestContextFilter : Bound request context to thread: org.apache.catalina.connector.RequestFacade@28d1a4b7
google.cloud.vision.v1.EntityAnnotation.locale : en
google.cloud.vision.v1.EntityAnnotation.description : Google
google.cloud.vision.v1.EntityAnnotation.bounding_poly : vertices {
x: 2
y: 2
}
vertices {
x: 267
y: 2
}
vertices {
x: 267
y: 89
}
vertices {
x: 2
y: 89
}
google.cloud.vision.v1.EntityAnnotation.description : Google
google.cloud.vision.v1.EntityAnnotation.bounding_poly : vertices {
x: 2
y: 2
}
vertices {
x: 267
y: 2
}
vertices {
x: 267
y: 89
}
vertices {
x: 2
y: 89
}
2017-10-27 15:15:32.062 DEBUG 28013 --- [nio-8080-exec-3] o.s.b.w.f.OrderedRequestContextFilter : Cleared thread-bound request context: org.apache.catalina.connector.RequestFacade@28d1a4b7
'Google API > Vision' 카테고리의 다른 글
Vision - 3. Athentication (0) | 2020.02.06 |
---|---|
Vision - 2. Make a Request (0) | 2020.02.06 |
Vision - 1. 시작하기 / Enable the API (0) | 2020.02.06 |